import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: Scaffold(
backgroundColor: Colors.grey,
appBar: AppBar(
leading: const Icon(Icons.task_alt_outlined),
title: Text('Flutter: Primeiros Passos'),
),
body: ListView(
children: [
Square(Colors.white, Colors.pink, Colors.cyan),
Square(Colors.red, Colors.purple, Colors.blue),
Square(Colors.pinkAccent, Colors.yellow, Colors.lightBlue),
Square(Colors.purpleAccent, Colors.white, Colors.green),
],
)
)
);
}
}
class Square extends StatelessWidget {
final Color cor1;
final Color cor2;
final Color cor3;
const Square(this.cor1, this.cor2, this.cor3, {Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsets.all(8.0),
child: Row(
children: [
Container(
color: cor1,
height: 140,
width: 110,
),
Container(
color: cor2,
height: 140,
width: 110,
),
Container(
color: cor3,
height: 140,
width: 110,
),
],
),
);
}
}
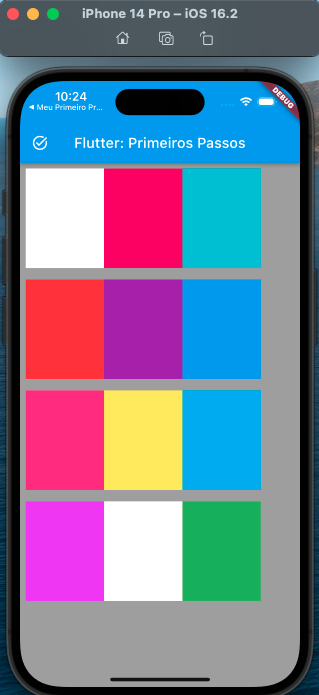